Sample Assembly Code: A Beginner's Guide to Low-Level Programming

Diving into the world of low-level programming can be both exciting and challenging. Sample assembly code serves as a foundational step for beginners looking to understand how computers execute instructions at the hardware level. By exploring assembly language, you gain insights into CPU operations, memory management, and the inner workings of software. This guide will walk you through the basics, providing a clear roadmap to start your journey in assembly programming, whether you're an aspiring developer or a tech enthusiast.
What is Assembly Language? (assembly language basics, low-level programming)
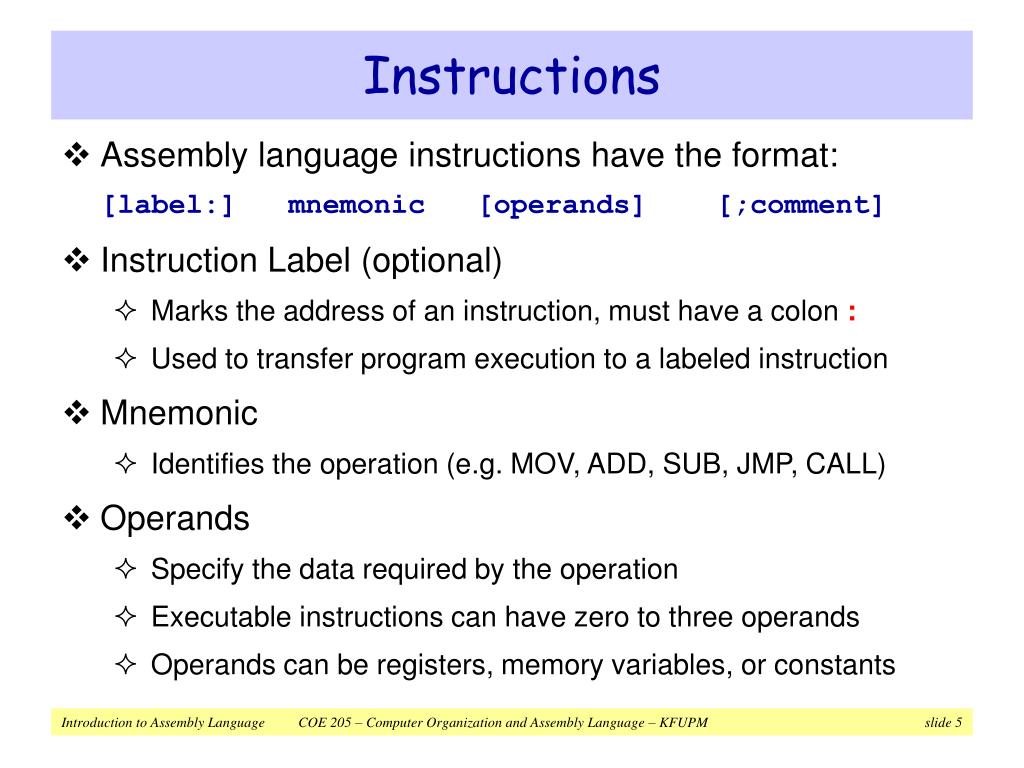
Assembly language is a low-level programming language that closely corresponds to machine code. Unlike high-level languages like Python or Java, assembly code is specific to the architecture of the processor it runs on. Each instruction in assembly directly maps to a machine code instruction, making it a powerful tool for optimizing performance and understanding hardware interactions.
Why Learn Assembly Code? (benefits of assembly programming, CPU architecture)
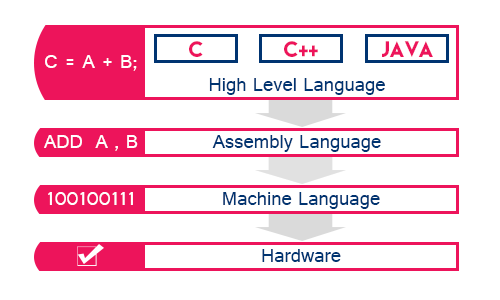
Learning assembly code offers several advantages, including:
- Deeper Understanding of Computing: Gain insights into how CPUs process instructions.
- Performance Optimization: Write highly efficient code for resource-constrained systems.
- Debugging Skills: Identify and fix low-level issues in software.
- Foundational Knowledge: Build a strong base for advanced programming and systems engineering.
Getting Started with Assembly Programming (assembly programming tutorial, beginner’s guide)

To begin your assembly programming journey, follow these steps:
- Choose an Assembly Language: Start with a widely used one like x86 or ARM, depending on your target hardware.
- Set Up Your Environment: Install an assembler and emulator (e.g., NASM and QEMU for x86).
- Learn Basic Instructions: Familiarize yourself with fundamental commands like MOV, ADD, and JMP.
- Write Your First Program: Start with simple tasks like printing text or performing arithmetic operations.
💡 Note: Assembly syntax varies by architecture, so ensure your learning resources match your chosen platform.
Sample Assembly Code Examples (assembly code examples, x86 assembly)
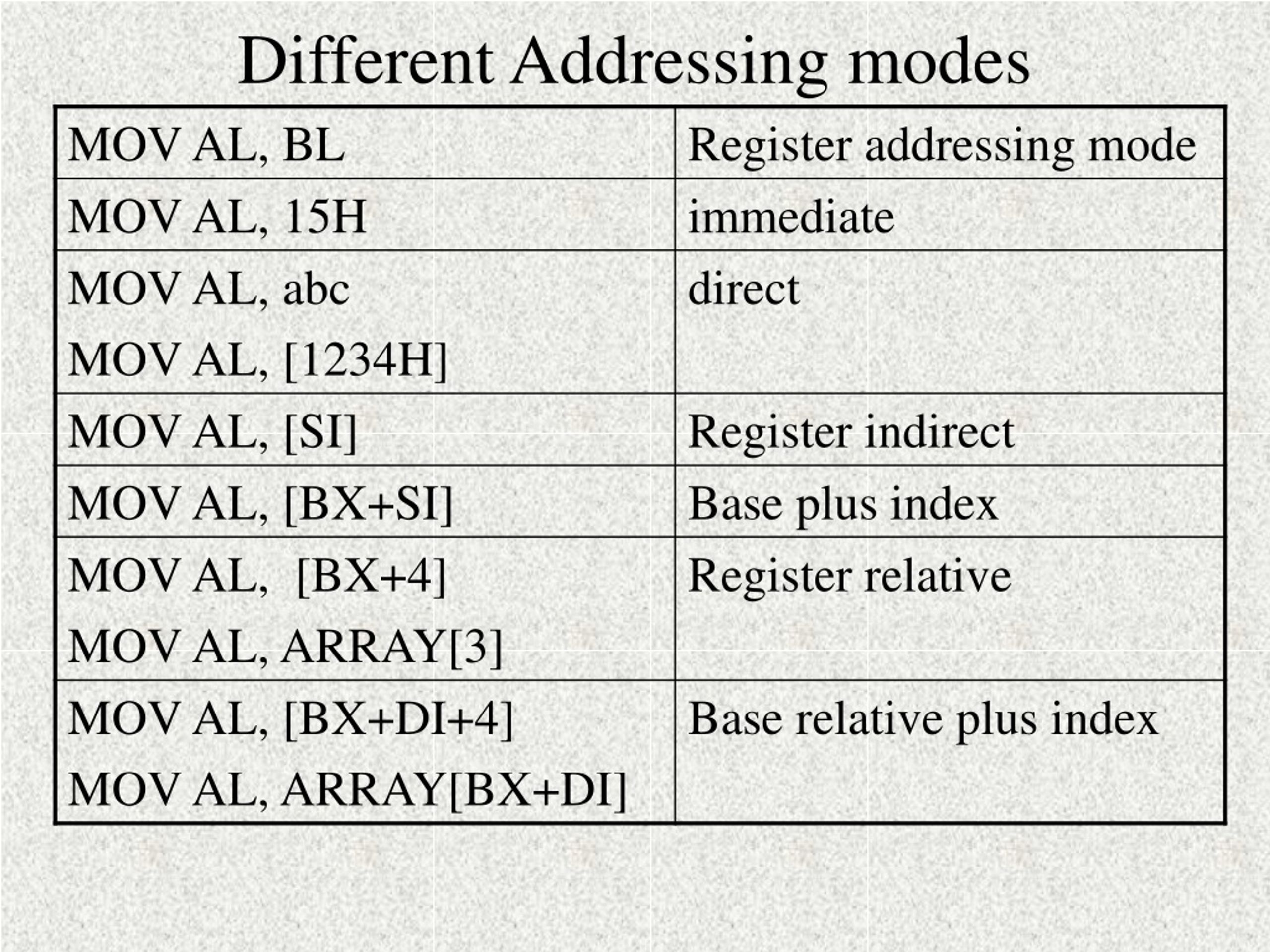
Here’s a simple x86 assembly code snippet to print “Hello, World!” to the console:
Code | Description |
---|---|
section .data msg db ‘Hello, World!’, 0xA len equ $ - msg |
Defines the data section with the message and its length. |
section .text global _start _start: mov eax, 4 mov ebx, 1 mov ecx, msg mov edx, len int 0x80 mov eax, 1 xor ebx, ebx int 0x80 |
Defines the code section to print the message and exit the program. |

Tips for Learning Assembly Code (assembly programming tips, learning assembly)
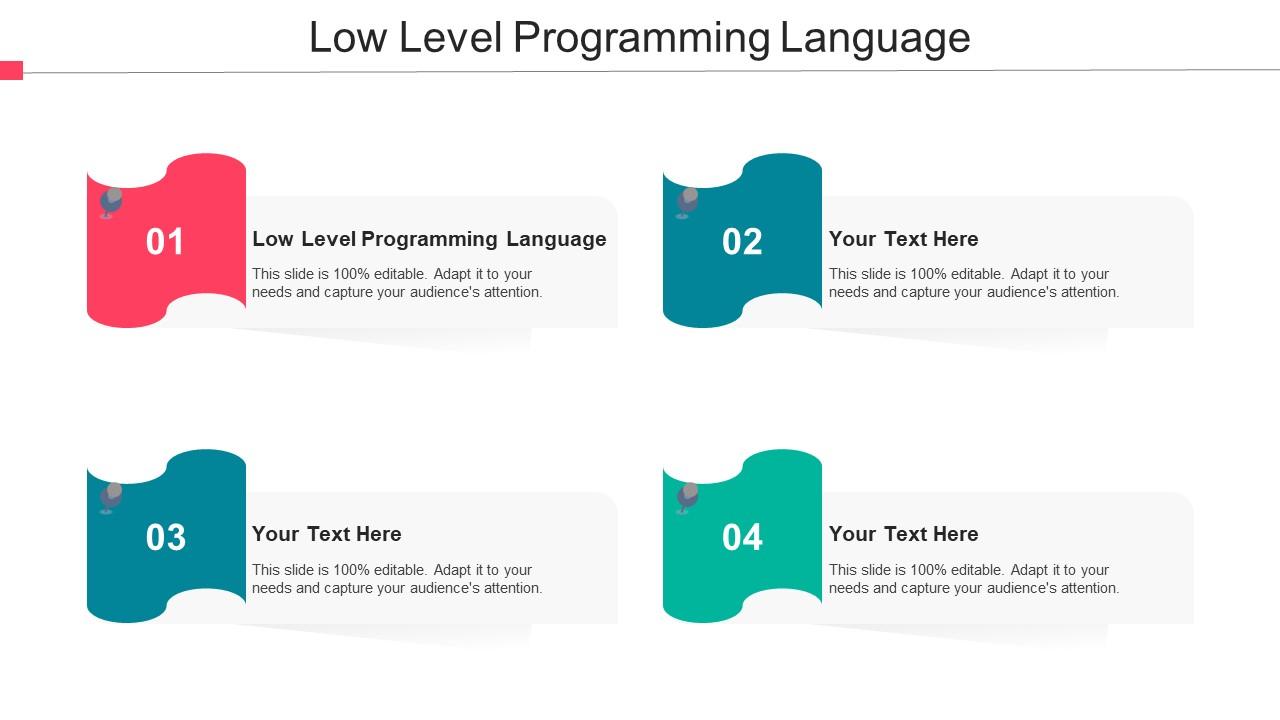
To master assembly programming, keep these tips in mind:
- Practice Regularly: Write and debug small programs to reinforce your skills.
- Read Documentation: Familiarize yourself with processor manuals and assembly references.
- Join Communities: Engage with forums and groups to learn from experienced programmers.
- Experiment with Projects: Apply your knowledge to real-world tasks like creating simple games or utilities.
Summary and Checklist (assembly programming checklist, summary)
Here’s a quick checklist to guide your assembly programming journey:
- Choose an assembly language and set up your environment.
- Learn basic instructions and write your first program.
- Practice regularly and experiment with projects.
- Join communities and read documentation for continuous learning.
Exploring sample assembly code is a rewarding experience that deepens your understanding of computing fundamentals. With patience and practice, you’ll gain the skills to write efficient, low-level programs. Whether you’re optimizing performance or debugging complex systems, assembly programming is a valuable skill in any developer’s toolkit. Start small, stay consistent, and enjoy the journey into the heart of computing.
What is assembly language used for?
+Assembly language is used for low-level programming, performance optimization, and understanding CPU architecture. It’s also essential for embedded systems and operating system development.
Is assembly language hard to learn?
+Assembly language can be challenging due to its low-level nature, but with consistent practice and the right resources, beginners can gradually master it.
Which assembly language should I start with?
+For beginners, x86 assembly is a popular choice due to its widespread use. ARM assembly is also a great option, especially for mobile and embedded systems.